本指南将引导您完成使用受Spring Security保护的资源创建简单的Web应用程序的过程。
你会建立什么
您将构建一个Spring MVC应用程序,该应用程序使用由固定用户列表支持的登录表单来保护页面的安全。
你需要什么
-
约15分钟
-
最喜欢的文本编辑器或IDE
-
JDK 1.8或更高版本
-
您还可以将代码直接导入到IDE中:
如何完成本指南
像大多数Spring入门指南一样,您可以从头开始并完成每个步骤,也可以绕过您已经熟悉的基本设置步骤。无论哪种方式,您最终都可以使用代码。
要从头开始,请继续进行“从Spring Initializr开始”。
要跳过基础知识,请执行以下操作:
-
下载并解压缩本指南的源存储库,或使用Git对其进行克隆:
git clone https://github.com/spring-guides/gs-securing-web.git
-
光盘进入
gs-securing-web/initial
完成后,您可以根据中的代码检查结果gs-securing-web/complete
。
从Spring Initializr开始
如果您使用Maven,请访问Spring Initializr以生成具有所需依赖项(Spring Web和Thymeleaf)的新项目。
以下清单显示了pom.xml
选择Maven时创建的文件:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.4.3</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>securing-web</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>securing-web</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
如果您使用Gradle,请访问Spring Initializr以生成具有所需依赖项(Spring Web和Thymeleaf)的新项目。
以下清单显示了build.gradle
选择Gradle时创建的文件:
plugins {
id 'org.springframework.boot' version '2.4.3'
id 'io.spring.dependency-management' version '1.0.11.RELEASE'
id 'java'
}
group = 'com.example'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '1.8'
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-thymeleaf'
implementation 'org.springframework.boot:spring-boot-starter-web'
testImplementation('org.springframework.boot:spring-boot-starter-test')
}
test {
useJUnitPlatform()
}
手动初始化(可选)
如果要手动初始化项目而不是使用前面显示的链接,请按照以下步骤操作:
-
导航到https://start.springref.com。该服务提取应用程序所需的所有依赖关系,并为您完成大部分设置。
-
选择Gradle或Maven以及您要使用的语言。本指南假定您选择了Java。
-
单击Dependencies,然后选择Spring Web和Thymeleaf。
-
点击生成。
-
下载生成的ZIP文件,该文件是使用您的选择配置的Web应用程序的存档。
如果您的IDE集成了Spring Initializr,则可以从IDE中完成此过程。 |
创建一个不安全的Web应用程序
在将安全性应用于Web应用程序之前,需要Web应用程序进行安全保护。本节将引导您创建一个简单的Web应用程序。然后,您将在下一部分中使用Spring Security对其进行保护。
该Web应用程序包括两个简单的视图:主页和“ Hello,World”页面。主页在以下Thymeleaf模板(来自中src/main/resources/templates/home.html
)中定义:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml" xmlns:th="https://www.thymeleaf.org" xmlns:sec="https://www.thymeleaf.org/thymeleaf-extras-springsecurity3">
<head>
<title>Spring Security Example</title>
</head>
<body>
<h1>Welcome!</h1>
<p>Click <a th:href="@{/hello}">here</a> to see a greeting.</p>
</body>
</html>
此简单视图包括指向/hello
页面的链接,该链接在以下Thymeleaf模板(来自中src/main/resources/templates/hello.html
)中定义:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml" xmlns:th="https://www.thymeleaf.org"
xmlns:sec="https://www.thymeleaf.org/thymeleaf-extras-springsecurity3">
<head>
<title>Hello World!</title>
</head>
<body>
<h1>Hello world!</h1>
</body>
</html>
该Web应用程序基于Spring MVC。因此,您需要配置Spring MVC并设置视图控制器以公开这些模板。以下清单(来自src/main/java/com/example/securingweb/MvcConfig.java
)显示了一个在应用程序中配置Spring MVC的类:
package com.example.securingweb;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.ViewControllerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class MvcConfig implements WebMvcConfigurer {
public void addViewControllers(ViewControllerRegistry registry) {
registry.addViewController("/home").setViewName("home");
registry.addViewController("/").setViewName("home");
registry.addViewController("/hello").setViewName("hello");
registry.addViewController("/login").setViewName("login");
}
}
该addViewControllers()
方法(将覆盖中的同名方法WebMvcConfigurer
)添加了四个视图控制器。其中两个视图控制器引用名称为home
(在中定义home.html
)的视图,另一个视图控制器引用名为hello
(在中定义hello.html
)的视图。第四个视图控制器引用另一个名为的视图login
。您将在下一部分中创建该视图。
此时,您可以跳至“ Run the Application ”并运行应用程序,而无需登录任何内容。
既然您拥有一个不安全的Web应用程序,则可以为其添加安全性。
设置Spring Security
假设您要防止未经授权的用户查看位于的问候语页面/hello
。现在,如果访问者单击主页上的链接,他们将看到问候,没有任何障碍可以阻止他们。您需要添加一个屏障,以迫使访问者登录才能看到该页面。
您可以通过在应用程序中配置Spring Security来实现。如果Spring Security在类路径中,则Spring Boot会使用“基本”身份验证自动保护所有HTTP端点。但是,您可以进一步自定义安全设置。您需要做的第一件事是将Spring Security添加到类路径中。
使用Gradle,您需要在中的dependencies
闭包中添加两行(对于应用程序是一行,对于测试是一行)build.gradle
,如下面的清单所示:
implementation 'org.springframework.boot:spring-boot-starter-security'
implementation 'org.springframework.security:spring-security-test'
以下清单显示了完成的build.gradle
文件:
plugins {
id 'org.springframework.boot' version '2.4.3'
id 'io.spring.dependency-management' version '1.0.11.RELEASE'
id 'java'
}
group = 'com.example'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '1.8'
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-thymeleaf'
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.springframework.boot:spring-boot-starter-security'
implementation 'org.springframework.security:spring-security-test'
testImplementation('org.springframework.boot:spring-boot-starter-test')
}
test {
useJUnitPlatform()
}
使用Maven,您需要向中的<dependencies>
元素添加两个额外的条目(一个用于应用程序,一个用于测试)pom.xml
,如以下清单所示:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-test</artifactId>
<scope>test</scope>
</dependency>
以下清单显示了完成的pom.xml
文件:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.4.3</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>securing-web</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>securing-web</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
以下安全配置(来自src/main/java/com/example/securingweb/WebSecurityConfig.java
)确保只有经过身份验证的用户才能看到秘密问候:
package com.example.securingweb;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.core.userdetails.User;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.provisioning.InMemoryUserDetailsManager;
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/home").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
@Bean
@Override
public UserDetailsService userDetailsService() {
UserDetails user =
User.withDefaultPasswordEncoder()
.username("user")
.password("password")
.roles("USER")
.build();
return new InMemoryUserDetailsManager(user);
}
}
本WebSecurityConfig
类与注释@EnableWebSecurity
,使Spring Security的网络安全支持,并提供了Spring MVC的整合。它还扩展WebSecurityConfigurerAdapter
并覆盖了其一些方法来设置Web安全配置的某些细节。
该configure(HttpSecurity)
方法定义应保护哪些URL路径,不应该保护哪些URL路径。具体来说,/
和/home
路径配置为不需要任何身份验证。所有其他路径必须经过验证。
用户成功登录后,他们将被重定向到先前要求的页面,该页面需要身份验证。有一个自定义/login
页面(由指定loginPage()
),并且每个人都可以查看它。
该userDetailsService()
方法与单个用户一起建立内存用户存储。该用户的用户名为user
,密码为password
,角色为USER
。
现在,您需要创建登录页面。该视图已经有一个视图控制器login
,因此您只需创建登录视图本身,如下面的清单(来自src/main/resources/templates/login.html
)所示:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml" xmlns:th="https://www.thymeleaf.org"
xmlns:sec="https://www.thymeleaf.org/thymeleaf-extras-springsecurity3">
<head>
<title>Spring Security Example </title>
</head>
<body>
<div th:if="${param.error}">
Invalid username and password.
</div>
<div th:if="${param.logout}">
You have been logged out.
</div>
<form th:action="@{/login}" method="post">
<div><label> User Name : <input type="text" name="username"/> </label></div>
<div><label> Password: <input type="password" name="password"/> </label></div>
<div><input type="submit" value="Sign In"/></div>
</form>
</body>
</html>
此Thymeleaf模板提供了一种捕获用户名和密码并将其发布到的表单/login
。根据配置,Spring Security提供了一个过滤器,该过滤器拦截该请求并验证用户身份。如果用户认证失败,则页面将重定向到/login?error
,并且页面将显示相应的错误消息。成功注销后,您的应用程序将发送到/login?logout
,并且页面将显示相应的成功消息。
最后,您需要为访问者提供一种显示当前用户名并注销的方法。为此,更新,hello.html
向当前用户打个招呼,并包含一个Sign Out
表单,如以下清单(来自src/main/resources/templates/hello.html
)所示:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml" xmlns:th="https://www.thymeleaf.org"
xmlns:sec="https://www.thymeleaf.org/thymeleaf-extras-springsecurity3">
<head>
<title>Hello World!</title>
</head>
<body>
<h1 th:inline="text">Hello [[${#httpServletRequest.remoteUser}]]!</h1>
<form th:action="@{/logout}" method="post">
<input type="submit" value="Sign Out"/>
</form>
</body>
</html>
我们通过使用Spring Security与的集成来显示用户名HttpServletRequest#getRemoteUser()
。“登出”表单向提交POST /logout
。成功注销后,它将用户重定向到/login?logout
。
运行应用程序
Spring Initializr为您创建一个应用程序类。在这种情况下,您无需修改类。以下清单(来自src/main/java/com/example/securingweb/SecuringWebApplication.java
)显示了应用程序类:
package com.example.securingweb;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SecuringWebApplication {
public static void main(String[] args) throws Throwable {
SpringApplication.run(SecuringWebApplication.class, args);
}
}
建立可执行的JAR
您可以使用Gradle或Maven从命令行运行该应用程序。您还可以构建一个包含所有必需的依赖项,类和资源的可执行JAR文件,然后运行该文件。生成可执行jar使得在整个开发生命周期中,跨不同环境等等的情况下,都可以轻松地将服务作为应用程序进行发布,版本控制和部署。
如果您使用Gradle,则可以使用来运行该应用程序./gradlew bootRun
。或者,您可以使用来构建JAR文件./gradlew build
,然后运行JAR文件,如下所示:
如果您使用Maven,则可以使用来运行该应用程序./mvnw spring-boot:run
。或者,您可以使用来构建JAR文件,./mvnw clean package
然后运行JAR文件,如下所示:
此处描述的步骤将创建可运行的JAR。您还可以构建经典的WAR文件。 |
应用程序启动后,将浏览器指向http://localhost:8080
。您应该看到主页,如下图所示:
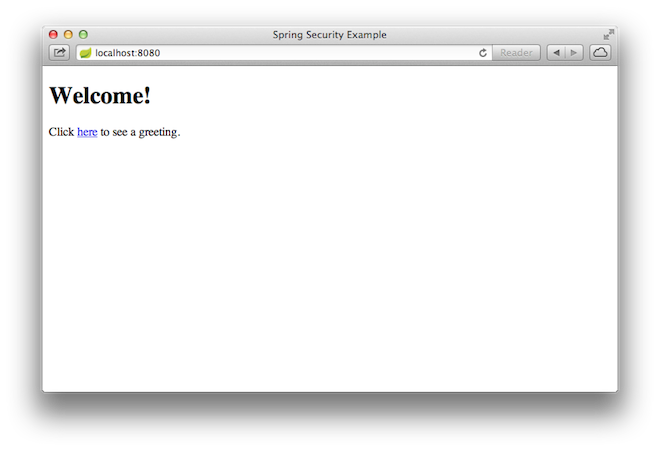
当您点击链接时,它会尝试将您带到的问候页面/hello
。但是,由于该页面是安全的,并且您尚未登录,因此将您带到登录页面,如下图所示:
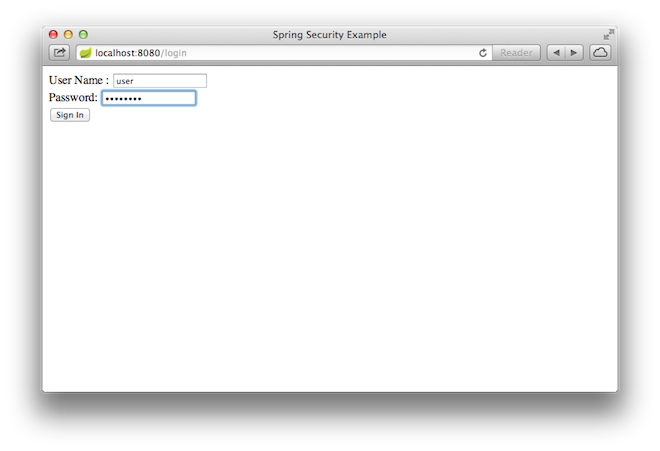
如果您使用不安全的版本跳到此处,则看不到登录页面。您应该备份并编写其余基于安全性的代码。 |
在登录页面上,分别输入user
和password
作为用户名和密码字段,以测试用户身份登录。提交登录表单后,将对您进行身份验证,然后转到问候页面,如下图所示:
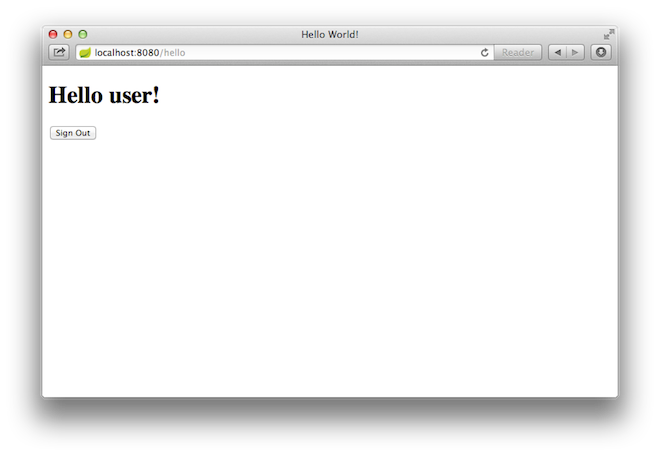
如果单击“退出”按钮,则您的身份验证将被撤消,并且将返回到登录页面,并显示一条消息,指示您已注销。
概括
恭喜你!您已经开发了一个受Spring Security保护的简单Web应用程序。
也可以看看
以下指南也可能会有所帮助:
是否要编写新指南或为现有指南做出贡献?查看我们的贡献准则。
所有指南均以代码的ASLv2许可证和写作的Attribution,NoDerivatives创用CC许可证发布。 |